List Iteration in Salesforce Lightning Web Component (LWC)
List Iteration In LWC
In the previous EPISODE, we have discussed ways of Data Binding In lightning web component.
In this EPISODE, we will discuss the ways of List Iteration in a lightning web component.
Before starting with this let's first understand when we need to Iterate the list in LWC? Alright !! So whenever we have a collection of data for example list of contact records, in such cases we need to iterate these list on HTML (template) and display the data on the screen may be in the form of the table etc.
It's similar to the <aura:iteration> in aura components.
So let's get started with the ways of List Iteration in LWC :
There are 2 ways of iterating your list in LWC templates :
1. For:Each
2. Iterator
What's the difference ??
Well, the meaning of both is quite similar but the purpose is different. Let's understand how :
1. For:Each :
This is a simple HTML template iterating way which is used when you simply want to iterate the list by providing a unique key value in the list.
The key here is the unique value to identify your record from the list.
These key will be helpful where you want to select the item from the list and post-process that particular record.
Let's understand from the syntax of for:each template :
SYNTAX :
-------------------------------------------------------
<template>
<template for:each={yourList} for:item="listVariable">
<div key={listVariable.UniqueValue}>
{listVariable.listItemLabel}
</div>
</template>
</template>
-------------------------------------------------------
In the above syntax {yourList} is your list to iterate for example contacts.
Then "listVariable" is the temporary variable set for your list it can be anything for example: for a list of contacts, the listVariable can be con.
Then key={listVariable.UniqueValue} is the unique value to identify the value from the list for example: it can be con.Id likewise.
Now let's cook some recipe to understand this better :
Follow the below steps :
STEP 1 :
Open Your VS Code and Create a Lightning Web Component called lwcForEach.
NOTE : If you want to start VS Code from scratch and Setup of local development then please check it out the previous EPISODE.
STEP 2 :
Write the code as below :
lwcForEach.html
-------------------------------------------------------
-------------------------------------------------------
In the above HTML template, you can see the same syntax was added with some extra tags like the title in <b> tag.
Then below that, we are iterating {contacts} with the list variable name con.
And for key we have used the {con.Id} from the list to identify the uniqueness of the list item.
And finally inside <div> we are defining what are the values we want from the list like we want to display Id, Name, Website.
Now let's take a look at .Js file to see what exactly {contacts} list contains in the client-side 😬.
lwcForEach.js
-------------------------------------------------------
-------------------------------------------------------
In the above JavaScript file we have just defined the sample hardcoded sample list.
Why not real contacts from Salesforce database? Relax !! kid this is just the start we are going to learn that in the upcoming lwc episode 😊.
For better UI I have added custom CSS. So we need to create the new css file with the name lwcForEach.css under the same component hierarchy.
lwcForEach.css
-------------------------------------------------------
-------------------------------------------------------
In the above CSS file I have added the simple CSS properties and added these classes in HTML tags for a better look.
NOTE : If you want to learn the CSS please check the W3School website for basic learnings.
I am super excited to preview this component.
STEP 3 :
For previewing this lightning web component I preferred two methods :
1. Adding this lightning component in lightning application just like we do in aura components
2. I believe in trying this new way of previewing this component with salesforce local development.
You can too !! Just run the below two commands in the terminal of VS code to start your personal local developement
command 1 :
-------------------------------------------------------
sfdx plugins:install @salesforce/lwc-dev-server
-------------------------------------------------------
command 2 :
-------------------------------------------------------
sfdx force:lightning:lwc:start
-------------------------------------------------------
and that's now you can display your preview localhost:3333 port in your browser.
Vs Code Restart recommended
Then just right-click on the lwcForEach component and select SFDX: Preview Component Locally

And finally, just the output will open in your browser as :
OUTPUT :
-------------------------------------------------------
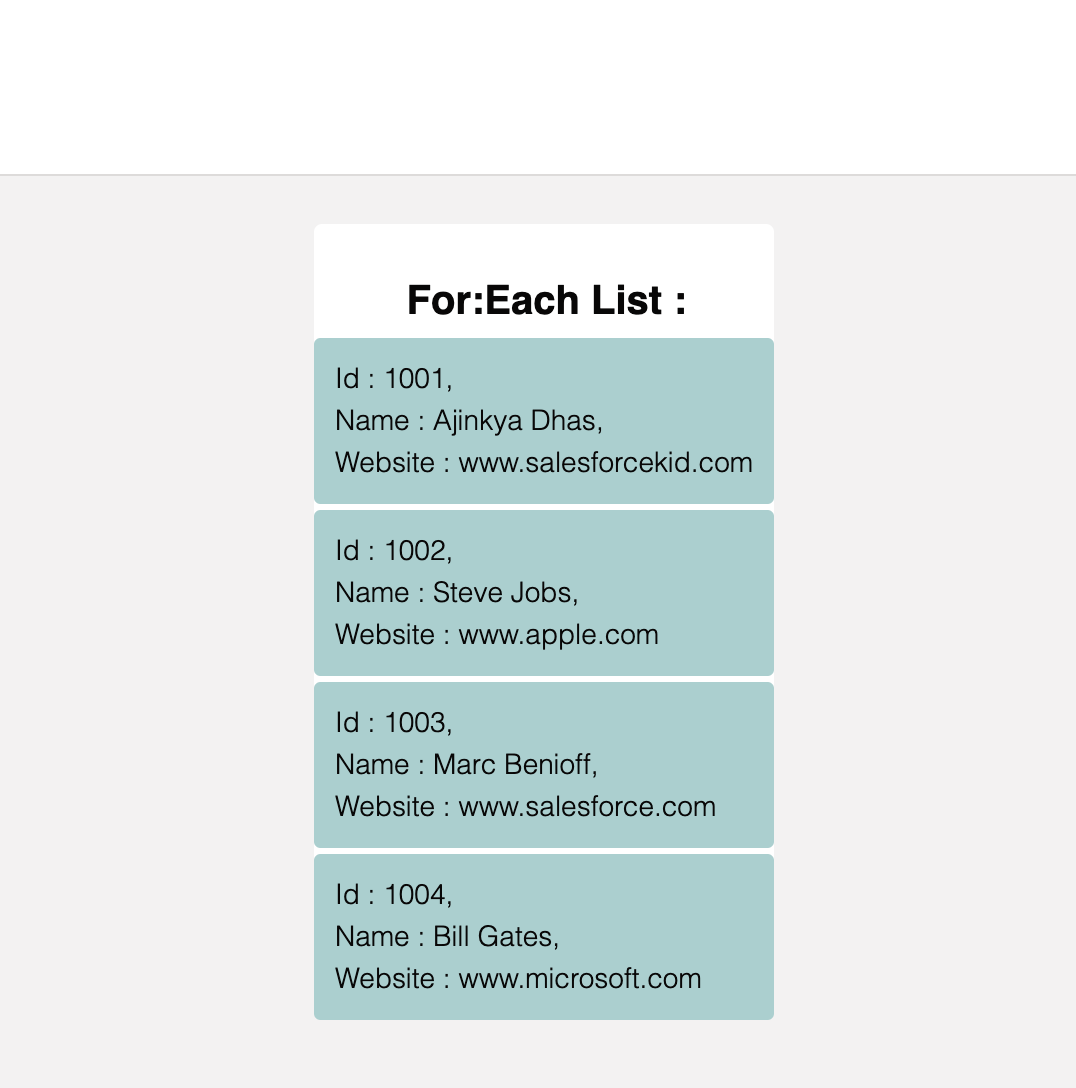
-------------------------------------------------------
Coool !! 😎 Right ?? Go...Go...Go...Kid 🏃♂️
Now let's take a look at another way of List Iteration by using Iterator.
2. Iterator :
This is an another way of iterating the list in HTML template.
The key difference from for:each is, In Iterator we can identify the first and last item from the list.
This can be used when you want to take any decision based on the first and last item from the list. the best example is pagination of a list where you can want to stop going forward or back based on the first and last page of the list.
Let's take a look at Syntax of Iterator :
SYNTAX :
-------------------------------------------------------
<template>
<template iterator:it={list}>
<div key={it.uniqueKey}>
<div if:true={it.first} class="doSomething">
</div>
{it.listItemlabel}
<div if:true={it.last} class="doSomething">
</div>
</div>
</template>
</template>
-------------------------------------------------------
In the above code {list} is the main list.
if:true={it.first} is the check for first item in the list.
class="doSomething" is the action taken on the first item in the list as here I have used the different class for the first item.
This can be other action as well as we can take different action by calling javascript
key={it.uniqueKey} is the unique key same as before to keep the item unique with this value in the list.
if:true={it.last} is to check for the last item in the list and take necessary action.
Let's understand by creating a new LWC Component for Iterator 😊.
Follow the below steps :
STEP 1 :
Create a new Lightning web component called lwcForIterator from VS Code.
STEP 2 :
Write the code as below :
lwcForIterator.html
-------------------------------------------------------
-------------------------------------------------------
In the above HTML template, you can see we have used the same pattern from the syntax.
Where as changeFirst is the class will be applied if the element is first from the div tag.
And changeLast is the last class will be applied if the element is last from the div tag.
Hence for the rest of the elements, these class will not be applied. We will have a great look when we preview the output.
lwcForIterator.js
-------------------------------------------------------
-------------------------------------------------------
We are using the same contacts list to iterate as we just need the list from javascript file.
Similar to the previous component we need to create the new CSS file called as lwcForIterator.css as below to provide the styling.
lwcForIterator.css
-------------------------------------------------------
-------------------------------------------------------
Now for preview just like before right-clicking on the component and select SFDX: Preview Component Locally.
And Hoolaaaa.......
OUTPUT :
-------------------------------------------------------
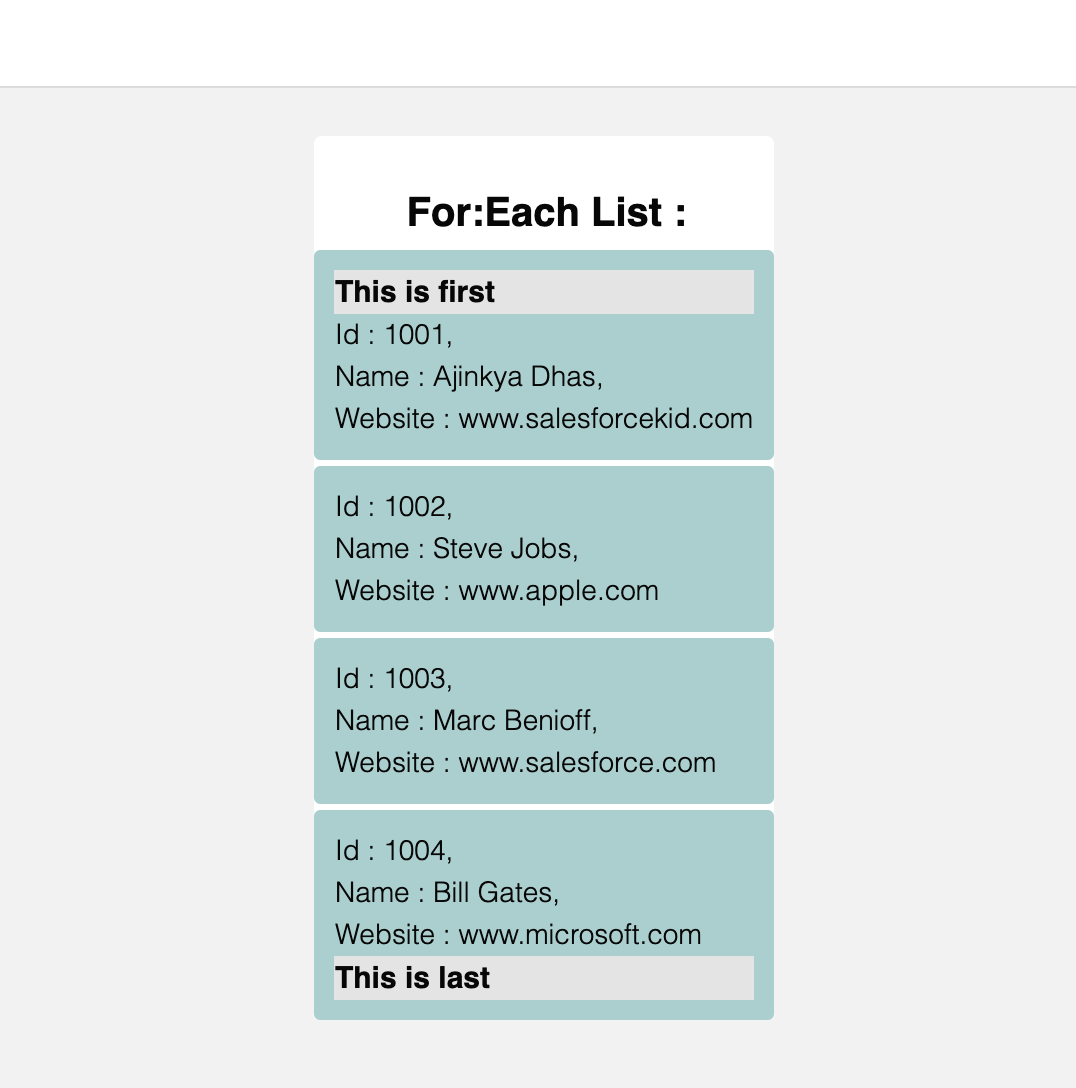
-------------------------------------------------------
Woooo...As you can see in the output The Div tag with the applied CSS is visible separately above the first element and below the last element.
This example also demonstrates that LWC component behaviour of checking the first and last element from the list.
In the Next EPISODE, we will discuss the Conditional Rendering in Salesforce Lightning Web Component 😊.
WOHOOO !! YOU HAVE JUST COMPLETED LIST ITERATION IN LIGHTNING WEB COMPONENT (LWC) EPISODE
If you like this salesforcekid learning platform please let me know in the Comment section...Also, Share with your salesforce folks wish you all
Happy Learning ☁️⚡️ (Learn. Help. Share.) 😊
<< PREVIOUS NEXT >>

It's similar to the <aura:iteration> in aura components.
So let's get started with the ways of List Iteration in LWC :
There are 2 ways of iterating your list in LWC templates :
1. For:Each
2. Iterator
What's the difference ??
Well, the meaning of both is quite similar but the purpose is different. Let's understand how :
1. For:Each :
This is a simple HTML template iterating way which is used when you simply want to iterate the list by providing a unique key value in the list.
The key here is the unique value to identify your record from the list.
These key will be helpful where you want to select the item from the list and post-process that particular record.
Let's understand from the syntax of for:each template :
SYNTAX :
-------------------------------------------------------
<template>
<template for:each={yourList} for:item="listVariable">
<div key={listVariable.UniqueValue}>
{listVariable.listItemLabel}
</div>
</template>
</template>
-------------------------------------------------------
In the above syntax {yourList} is your list to iterate for example contacts.
Then "listVariable" is the temporary variable set for your list it can be anything for example: for a list of contacts, the listVariable can be con.
Then key={listVariable.UniqueValue} is the unique value to identify the value from the list for example: it can be con.Id likewise.
Now let's cook some recipe to understand this better :
Follow the below steps :
STEP 1 :
Open Your VS Code and Create a Lightning Web Component called lwcForEach.
NOTE : If you want to start VS Code from scratch and Setup of local development then please check it out the previous EPISODE.
STEP 2 :
Write the code as below :
lwcForEach.html
-------------------------------------------------------
<template>
<lightning-card class="myfont">
<b class="myTitle">For:Each List :</b>
<template for:each={contacts} for:item="con">
<div key={con.Id} class="mypad">
Id : {con.Id},<br/>
Name : {con.Name},<br/>
Website : {con.Website}
</div>
</template>
</lightning-card>
</template>
In the above HTML template, you can see the same syntax was added with some extra tags like the title in <b> tag.
Then below that, we are iterating {contacts} with the list variable name con.
And for key we have used the {con.Id} from the list to identify the uniqueness of the list item.
And finally inside <div> we are defining what are the values we want from the list like we want to display Id, Name, Website.
Now let's take a look at .Js file to see what exactly {contacts} list contains in the client-side 😬.
lwcForEach.js
-------------------------------------------------------
import { LightningElement } from 'lwc';
export default class LwcForEach extends LightningElement {
//This contacts is our list for this demo
contacts = [
{
Id : '1001',
Name : 'Ajinkya Dhas',
Website : 'www.salesforcekid.com'
},
{
Id : '1002',
Name : 'Steve Jobs',
Website : 'www.apple.com'
},
{
Id : '1003',
Name : 'Marc Benioff',
Website : 'www.salesforce.com'
},
{
Id : '1004',
Name : 'Bill Gates',
Website : 'www.microsoft.com'
}
];
}
In the above JavaScript file we have just defined the sample hardcoded sample list.
Why not real contacts from Salesforce database? Relax !! kid this is just the start we are going to learn that in the upcoming lwc episode 😊.
For better UI I have added custom CSS. So we need to create the new css file with the name lwcForEach.css under the same component hierarchy.
lwcForEach.css
-------------------------------------------------------
.myfont{
font-family: sans-serif;
font-weight: lighter;
font-size: 14px;
}
.mypad{
padding: 10px;
background-color : #aacfcf;
margin-top: 3px;
border-radius: 0.2rem;
}
.myTitle{
font-size: 20px;
font-weight: bold;
position: relative;
left: 20%;
}
In the above CSS file I have added the simple CSS properties and added these classes in HTML tags for a better look.
NOTE : If you want to learn the CSS please check the W3School website for basic learnings.
I am super excited to preview this component.
STEP 3 :
For previewing this lightning web component I preferred two methods :
1. Adding this lightning component in lightning application just like we do in aura components
2. I believe in trying this new way of previewing this component with salesforce local development.
You can too !! Just run the below two commands in the terminal of VS code to start your personal local developement
command 1 :
-------------------------------------------------------
sfdx plugins:install @salesforce/lwc-dev-server
-------------------------------------------------------
command 2 :
-------------------------------------------------------
sfdx force:lightning:lwc:start
-------------------------------------------------------
and that's now you can display your preview localhost:3333 port in your browser.
Vs Code Restart recommended
Then just right-click on the lwcForEach component and select SFDX: Preview Component Locally

OUTPUT :
-------------------------------------------------------
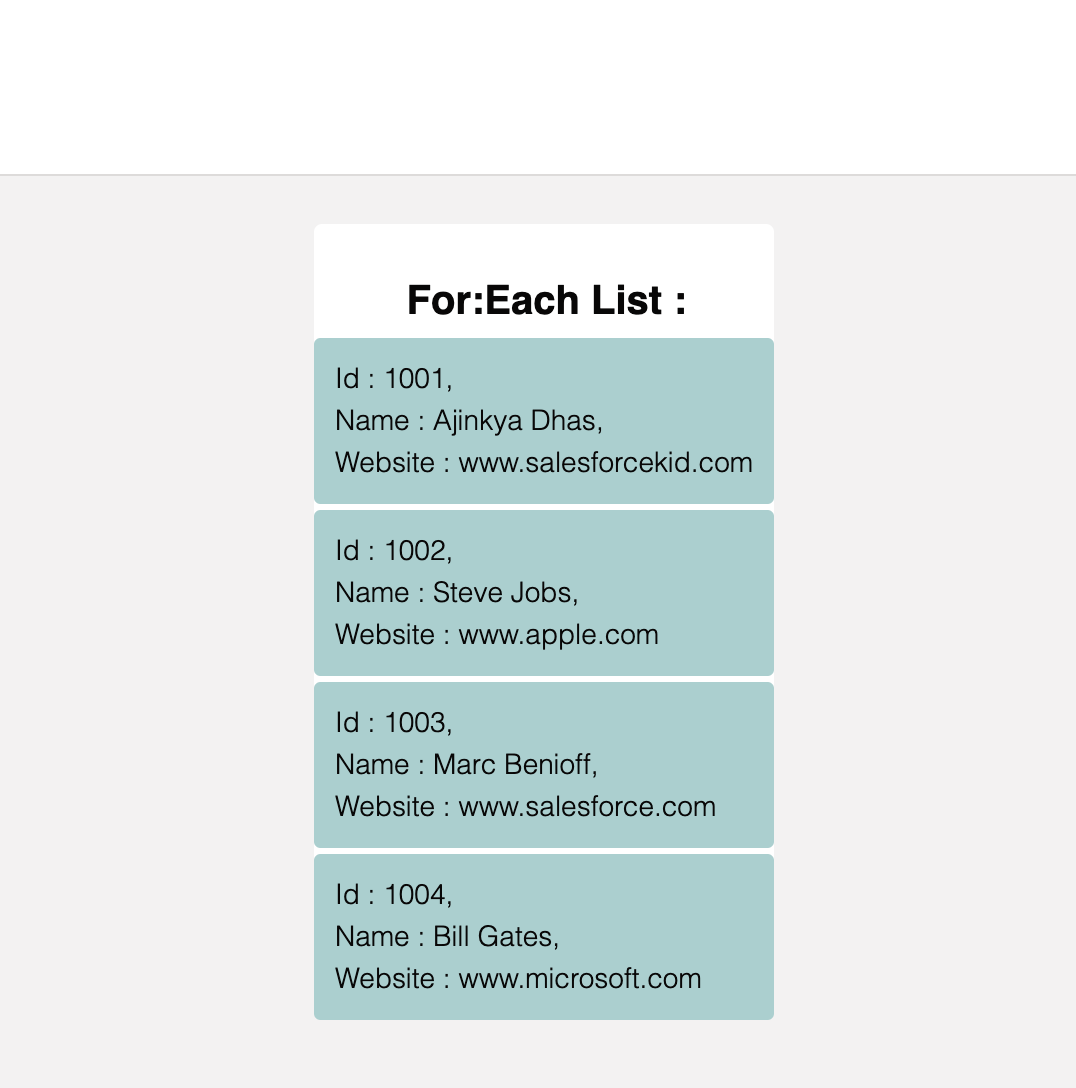
-------------------------------------------------------
Coool !! 😎 Right ?? Go...Go...Go...Kid 🏃♂️
Now let's take a look at another way of List Iteration by using Iterator.
2. Iterator :
This is an another way of iterating the list in HTML template.
The key difference from for:each is, In Iterator we can identify the first and last item from the list.
This can be used when you want to take any decision based on the first and last item from the list. the best example is pagination of a list where you can want to stop going forward or back based on the first and last page of the list.
Let's take a look at Syntax of Iterator :
SYNTAX :
-------------------------------------------------------
<template>
<template iterator:it={list}>
<div key={it.uniqueKey}>
<div if:true={it.first} class="doSomething">
</div>
{it.listItemlabel}
<div if:true={it.last} class="doSomething">
</div>
</div>
</template>
</template>
-------------------------------------------------------
In the above code {list} is the main list.
if:true={it.first} is the check for first item in the list.
class="doSomething" is the action taken on the first item in the list as here I have used the different class for the first item.
This can be other action as well as we can take different action by calling javascript
key={it.uniqueKey} is the unique key same as before to keep the item unique with this value in the list.
if:true={it.last} is to check for the last item in the list and take necessary action.
Let's understand by creating a new LWC Component for Iterator 😊.
Follow the below steps :
STEP 1 :
Create a new Lightning web component called lwcForIterator from VS Code.
STEP 2 :
Write the code as below :
lwcForIterator.html
-------------------------------------------------------
<template>
<lightning-card class="myfont">
<b class="myTitle">For:Each List :</b>
<template iterator:it={contacts}>
<div key={it.value.Id} class="mypad">
<div if:true={it.first} class="changeFirst">
This is first
</div>
Id : {it.value.Id},<br/>
Name : {it.value.Name},<br/>
Website : {it.value.Website}
<div if:true={it.last} class="changeLast">
This is last
</div>
</div>
</template>
</lightning-card>
</template>
In the above HTML template, you can see we have used the same pattern from the syntax.
Where as changeFirst is the class will be applied if the element is first from the div tag.
And changeLast is the last class will be applied if the element is last from the div tag.
Hence for the rest of the elements, these class will not be applied. We will have a great look when we preview the output.
lwcForIterator.js
-------------------------------------------------------
import { LightningElement } from 'lwc';
export default class LwcForIterator extends LightningElement {
//This contacts is our list for this demo
contacts = [
{
Id : '1001',
Name : 'Ajinkya Dhas',
Website : 'www.salesforcekid.com'
},
{
Id : '1002',
Name : 'Steve Jobs',
Website : 'www.apple.com'
},
{
Id : '1003',
Name : 'Marc Benioff',
Website : 'www.salesforce.com'
},
{
Id : '1004',
Name : 'Bill Gates',
Website : 'www.microsoft.com'
}
];
}
We are using the same contacts list to iterate as we just need the list from javascript file.
Similar to the previous component we need to create the new CSS file called as lwcForIterator.css as below to provide the styling.
lwcForIterator.css
-------------------------------------------------------
.myfont{
font-family: sans-serif;
font-weight: lighter;
font-size: 14px;
}
.mypad{
padding: 10px;
background-color : #aacfcf;
margin-top: 3px;
border-radius: 0.2rem;
}
.myTitle{
font-size: 20px;
font-weight: bold;
position: relative;
left: 20%;
}
.changeFirst{
font-weight: bold;
font-size: 15px;
background-color: #e4e4e4;
}
.changeLast{
font-weight: bold;
font-size: 15px;
background-color: #e4e4e4;
}
Now for preview just like before right-clicking on the component and select SFDX: Preview Component Locally.
And Hoolaaaa.......
OUTPUT :
-------------------------------------------------------
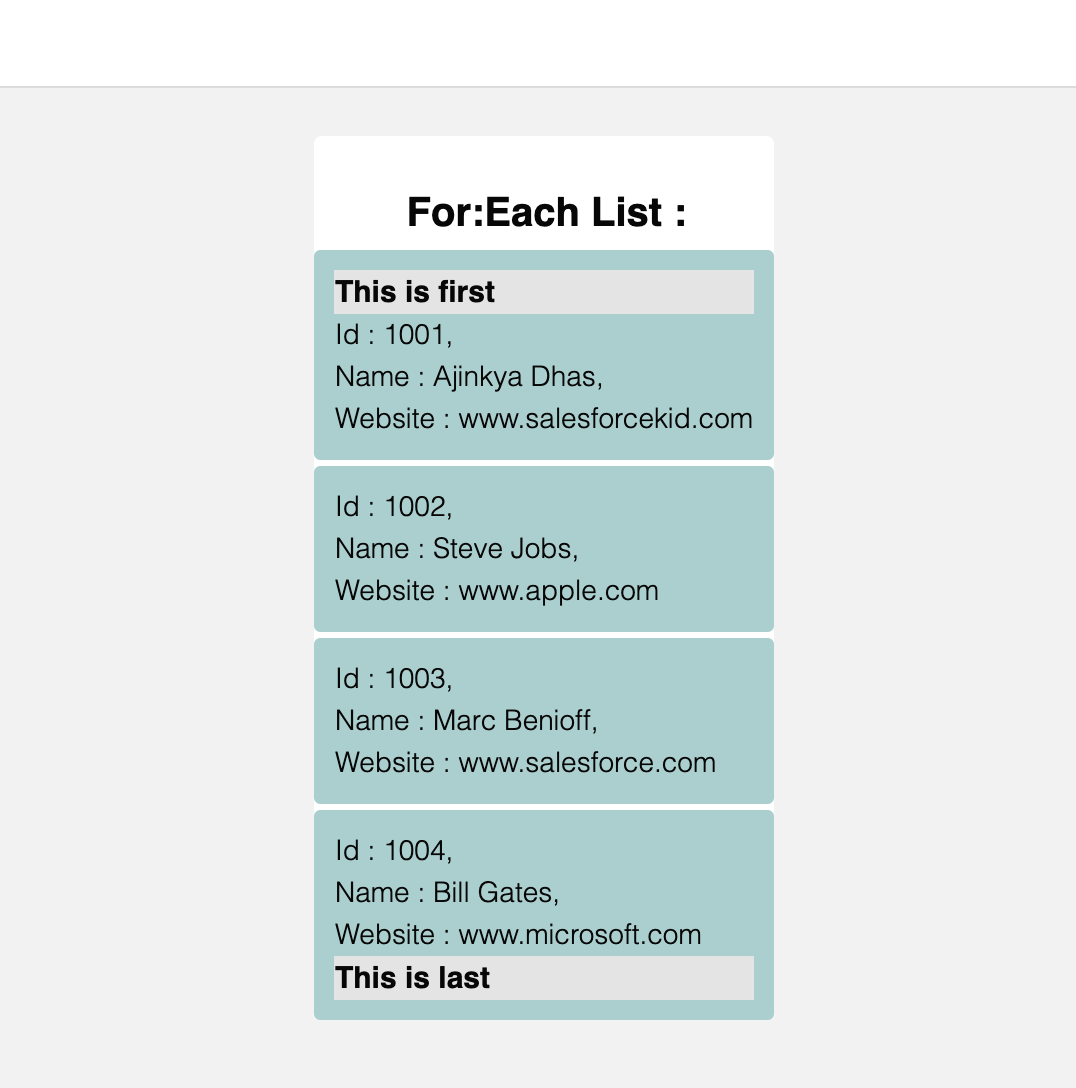
-------------------------------------------------------
Woooo...As you can see in the output The Div tag with the applied CSS is visible separately above the first element and below the last element.
This example also demonstrates that LWC component behaviour of checking the first and last element from the list.
In the Next EPISODE, we will discuss the Conditional Rendering in Salesforce Lightning Web Component 😊.
WOHOOO !! YOU HAVE JUST COMPLETED LIST ITERATION IN LIGHTNING WEB COMPONENT (LWC) EPISODE
If you like this salesforcekid learning platform please let me know in the Comment section...Also, Share with your salesforce folks wish you all
Happy Learning ☁️⚡️ (Learn. Help. Share.) 😊
<< PREVIOUS NEXT >>

List Iteration in Salesforce Lightning Web Component (LWC)
Reviewed by
on
Rating:

Nice information :)
ReplyDeleteThanks 😊
DeleteGreat Article
ReplyDeleteMass Compare Multiple Profiles
I am very much impressed with the simplicity of this tutorial. Thanks so much.
ReplyDeleteVery informative blog on Salesforce Development Services! Additionally, Tech9logy Creators is also one of the top-rated Salesforce Development Companies in India with over 10 years of experience. Opt for our Salesforce Development Services to bring the best out of your CRM investment!
ReplyDelete